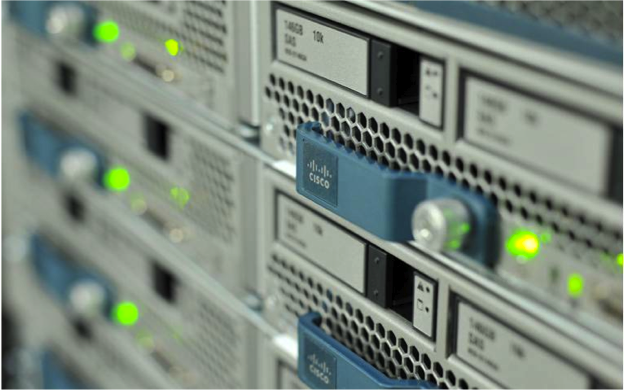
Add VLAN in UCS with PowerShell
Mostly I created VLANs in UCS with the GUI, but how do we do this in PowerShell?
When I have to create a lot of new VLANs, I want to do this in CLI 😎
The weird thing when starting with Cisco PowerShell Module is that you have to insert your credentials on nearly every command.
#Load Cisco UCS PS Module Import-Module CiscoUcsPS #Creds $ucsUserName = "myuser" $ucsPassword = "myp@ssword" $ucsSysName1 = "my-ucs-manager" # The UCSM connection requires a PSCredential to login, so we must convert our plain text password to make an object $ucsPassword = ConvertTo-SecureString -String $ucsPassword -AsPlainText -Force $cred = new-object -typename System.Management.Automation.PSCredential -argumentlist $ucsUserName, $ucsPassword ######################## # Authenticate to UCSM # ######################## $handle1 = Connect-Ucs $ucsSysName1 -NotDefault -Credential $cred #Define Variables $vlanid = 229 $vlname = "229-MyNewVlan" $vlangroup = "ESX-DMZ" $macpool = "My-MAC-Pool" $qpol = "ESX-Gold" $vnictmpdmzA = "DMZ-Data-FabA" $vnictmpdmzB = "DMZ-Data-FabB" #Create VLAN Global $handle1 = Connect-Ucs $ucsSysName1 -NotDefault -Credential $cred Get-UcsLanCloud | Add-UcsVlan -CompressionType "included" -DefaultNet "no" -Id $vlanid -McastPolicyName "" -Name $vlname -PolicyOwner "local" -PubNwName "" -Sharing "none" #Add VLAN to Group Start-UcsTransaction -Ucs $handle1 $mo = Get-UcsLanCloud -Ucs $handle1 | Add-UcsFabricNetGroup -ModifyPresent -Descr "" -NativeNet "" -PolicyOwner "local" -Type "mgmt" -Name $vlangroup $mo_1 = $mo | Add-UcsFabricPooledVlan -ModifyPresent -Name $vlname Complete-UcsTransaction -Ucs $handle1 #Add to Template FAB A Start-UcsTransaction -Ucs $handle1 $mo = Get-UcsOrg -Ucs $handle1 -Level root | Add-UcsVnicTemplate -ModifyPresent -Descr "" -IdentPoolName $macpool -Mtu 1500 -NwCtrlPolicyName "Enable-CDP" -PinToGroupName "" -PolicyOwner "local" -QosPolicyName $qpol -StatsPolicyName "default" -SwitchId "A" -TemplType "updating-template" -Name $vnictmpdmzA -XtraProperty @{CdnSource="vnic-name"; RedundancyPairType="none"; PeerRedundancyTemplName=""; AdminCdnName=""; } $mo_1 = $mo | Add-UcsVnicInterface -ModifyPresent -DefaultNet "no" -Name $vlname Complete-UcsTransaction #Add to Template FAB B Start-UcsTransaction -Ucs $handle1 $mo = Get-UcsOrg -Ucs $handle1 -Level root | Add-UcsVnicTemplate -ModifyPresent -Descr "" -IdentPoolName $macpool -Mtu 1500 -NwCtrlPolicyName "Enable-CDP" -PinToGroupName "" -PolicyOwner "local" -QosPolicyName $qpol -StatsPolicyName "default" -SwitchId "B" -TemplType "updating-template" -Name $vnictmpdmzB -XtraProperty @{CdnSource="vnic-name"; RedundancyPairType="none"; PeerRedundancyTemplName=""; AdminCdnName=""; } $mo_1 = $mo | Add-UcsVnicInterface -ModifyPresent -DefaultNet "no" -Name $vlname Complete-UcsTransaction -Ucs $handle1
Please, check out and write a comment!
Scared? Try first in a emulator to feel more confident.
4 thoughts on “Add VLAN in UCS with PowerShell”
Thanks for your marvelous posting! I actually enjoyed reading
it, you’re a great author. I will ensure that I bookmark your blog and will often come back from now on. I want to encourage continue your great work, have a nice
day!
How would you add a vlan GROUP to a vnic template?
Have a look at line 43-47
Am I missing it? that looks like adding a VLAN to a vnic template, but I want to add a vlan GROUP to a vnic template. Thanks!